Typealias vs. inline value class
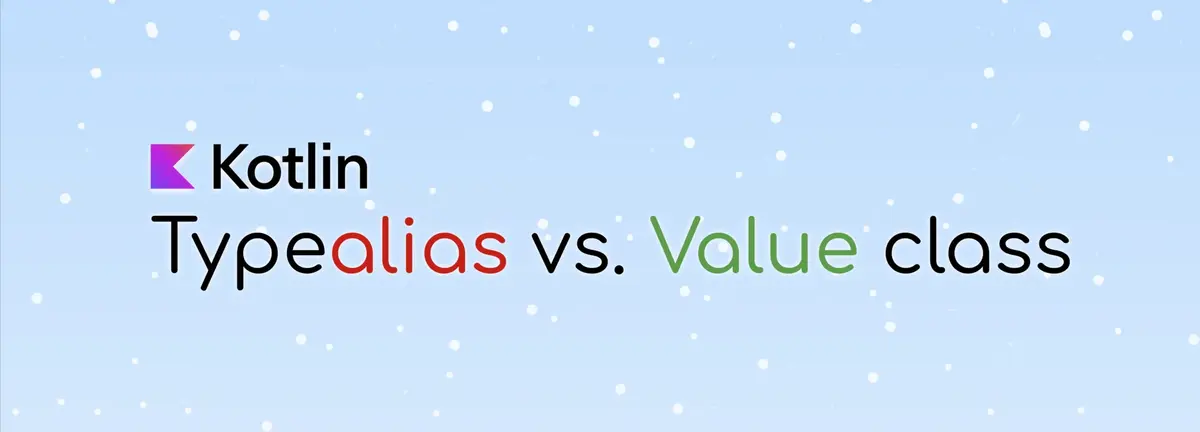
Normally, Typealias
and inline value class
(which I’ll refer to as value class
throughout this text) are utilized for different purposes. However, developers might occasionally use one for the other.
Let’s explore some examples and clarify the differences.
Typealias
kotlinlang.org does a pretty good job explaining Typealias
:
Type aliases provide alternative names for existing types. If the type name is too long you can introduce a different shorter name and use the new one instead.
For instance, it’s often tempting to shrink collection types:
|
|
You can have new names for inner and nested classes:
|
|
Inline value class
Let’s see how kotlinlang.org defines a value class
:
Sometimes it is useful to wrap a value in a class to create a more domain-specific type. Kotlin introduces a special kind of class called an inline class. Inline classes are a subset of value-based classes. They don’t have an identity and can only hold values.
To declare a value class
, use the value
modifier before the name of the class:
|
|
A value class
must have a single property initialized in the primary constructor. At runtime, instances of the value class
will be represented using this single property:
|
|
Use cases
Let’s consider the following simple data model Person
:
|
|
If you’re wondering how to ensure that the correct arguments are passed for similarly typed parameters like name
and family
, or age
and height
, Kotlin’s answer is the value class
:
|
|
Therefore, the caller needs to use the correct argument type for each corresponding parameter, as follows:
|
|
Using typealias
in this scenario is not a solution to the aforementioned problem:
|
|
Because no rule is enforced at the call site:
|
|
typealias Name = String
and use Name
in your code, the Kotlin compiler always expands it to String
.If you’d like to learn more about value class
, you can check here.